Assignment 1: Evergreen Field Trip (20 Points)
Chris Tralie
Due Monday 2/30/2020
The purpose of this assignment is two-fold:
- To get students practice with printing out properly formatted text output to the console
- To get students to model a small problem by hand and then solve it with the computer
EvergreenArt.java
for part 1 and FieldTrip.java
for part 2. When you are finished, you should submit both of these files to canvas. Please also submit answers to the following as a comment on Canvas:
- Approximately how many hours it took you to finish this assignment (I will not judge you for this at all...I am simply using it to gauge if the assignments are too easy or hard)
- Your overall impression of the assignment. Did you love it, hate it, or were you neutral? One word answers are fine, but if you have any suggestions for the future let me know.
- Any other concerns that you have. For instance, if you have a bug that you were unable to solve but you made progress, write that here. The more you articulate the problem the more partial credit you will receive (fine to leave this blank)
Part 1: Text Art
Edit the file EvergreenArt.java
so that when it's run, it prints out the following text, which makes a little "text picture" that looks like an evergreen tree:
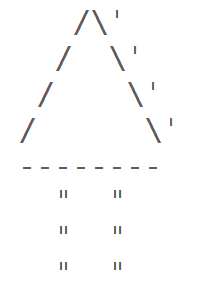
Tips
- Watch out for your special characters that need "escape sequences"! This includes double quotes and backslashes.
Part 2: Field Trip Logistics
A group of Ursinus students wants to take a trip to The Poconos. The school wants students to use as many full buses as they can to hold the students, and anyone who's leftover should take a van. You should edit FieldTrip.java
so that it takes as input the number of people going on the trip, the capacity of each bus, and the capacity of each van. Nobody should be left behind! So even if the last van isn't completely full, you need to count it.
As an example, suppose 87 students want to go on a field trip, a bus holds 10 people, and a van holds 4 people (yes, I know, these are small buses and vans, but they are valid for this problem). Then the most buses we can fill is 8 buses, but there are still 7 people leftover. We need 2 vans to hold those 7 people.
Tips
- If you use the
int
type, then division is truncated. So, for example,10 / 4
is 2. -
The modulus operator, %, may come in handy here. This gives the remainder after division. So, for example,
13 % 3
is 1. -
The
Math.ceil()
, the so-called "ceiling function," is useful for always rounding up. So, for example,Math.ceil(7.0/3.0)
is 3, even though 2.3333 normally rounds down to 2. This should help you for the leftover people with the vans. Note that you may have toint
values todouble
values before doing division, so that the decimal isn't completely dropped before trying to take the ceiling. For instance,Math.ceil(7/3)
is actually 2, not 3, butMath.ceil((double)7/(double)3)
is 3. You can test this out yourself in Java.